Pointers And Memory - Contents
A D V E R T I S E M E N T
In the simplest "pass by value" or "value parameter" scheme, each function has separate,
local memory and parameters are copied from the caller to the callee at the moment of the
function call. But what about the other direction? How can the callee communicate back
to its caller? Using a "return" at the end of the callee to copy a result back to the caller
works for simple cases, but does not work well for all situations. Also, sometimes
copying values back and forth is undesirable. "Pass by reference" parameters solve all of
these problems.
For the following discussion, the term "value of interest" will be a value that the caller
and callee wish to communicate between each other. A reference parameter passes a
pointer to the value of interest instead of a copy of the value of interest. This technique
uses the sharing property of pointers so that the caller and callee can share the value of
interest.
Bill Gates Example
Suppose functions A() and B() both do computations involving Bill Gates' net worth
measured in billions of dollars — the value of interest for this problem. A() is the main
function and its stores the initial value (about 55 as of 1998). A() calls B() which tries to
add 1 to the value of interest
Bill Gates By Value
Here is the code and memory drawing for a simple, but incorrect implementation where
A() and B() use pass by value. Three points in time, T1, T2, and T3 are marked in the
code and the state of memory is shown for each state...
void B(int worth) {
worth = worth + 1;
// T2
}
void A() {
int netWorth;
netWorth = 55; // T1
B(netWorth);
// T3 -- B() did not change netWorth
}
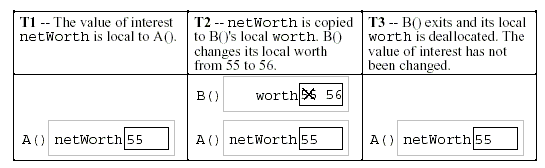
B() adds 1 to its local worth copy, but when B() exits, worth is deallocated, so
changing it was useless. The value of interest, netWorth, rests unchanged the whole
time in A()'s local storage. A function can change its local copy of the value of interest,
but that change is not reflected back in the original value. This is really just the old
"independence" property of local storage, but in this case it is not what is wanted
Vector allows us to view it's insides
using an Enumerator ; a class to go through objects. It is very useful to
first be able to look what you're looking for, and only later decide whether you'd like to
remove it or not. A sample program that uses java.util.Vector for it's
storage follows.
By Reference:
The reference solution to the Bill Gates problem is to use a single netWorth variable
for the value of interest and never copy it. Instead, each function can receives a pointer to
netWorth. Each function can see the current value of netWorth by dereferencing its
pointer. More importantly, each function can change the net worth — just dereference
the pointer to the centralized netWorth and change it directly. Everyone agrees what
the current value of netWorth because it exists in only one place — everyone has a
pointer to the one master copy. The following memory drawing shows A() and B()
functions changed to use "reference" parameters. As before, T1, T2, and T3 correspond to
points in the code (below), but you can study the memory structure without looking at the
code yet
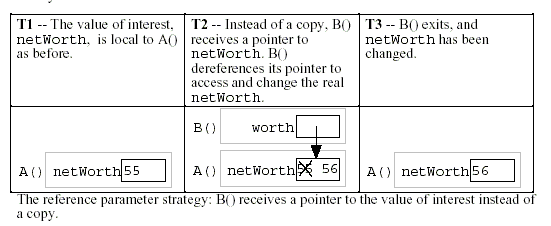
Passing By Reference:
Here are the steps to use in the code to use the pass-by-reference strategy
- Have a single copy of the value of interest. The single "master" copy.
- Pass pointers to that value to any function which wants to see or change
the value.
- Functions can dereference their pointer to see or change the value of
interest.
- Functions must remember that they do not have their own local copies. If
they dereference their pointer and change the value, they really are
changing the master value. If a function wants a local copy to change
safely, the function must explicitly allocate and initialize such a local
copy.
Syntax:
The syntax for by reference parameters in the C language just uses pointer operations on
the parameters
- Suppose a function wants to communicate about some value of interest —
int or float or struct fraction.
- The function takes as its parameter a pointer to the value of interest — an
int* or float* or struct fraction*. Some programmers will
add the word "ref" to the name of a reference parameter as a reminder that
it is a reference to the value of interest instead of a copy.
- .At the time of the call, the caller computes a pointer to the value of interest
and passes that pointer. The type of the pointer (pointer to the value of
interest) will agree with the type in (2) above. If the value of interest is
local to the caller, then this will often involve a use of the & operator
(Section 1).
- When the callee is running, if it wishes to access the value of interest, it
must dereference its pointer to access the actual value of interest.
Typically, this equates to use of the dereference operator (*) in the
function to see the value of interest.
Bill Gates By Reference:
Here is the Bill Gates example written to use reference parameters. This code now
matches the by-reference memory drawing above.
// B() now uses a reference parameter -- a pointer to
// the value of interest. B() uses a dereference (*) on the
// reference parameter to get at the value of interest.
void B(int* worthRef) { // reference parameter
*worthRef = *worthRef + 1; // use * to get at value of interest
// T2
}
void A() {
int netWorth;
netWorth = 55; // T1 -- the value of interest is local to A()
B(&netWorth); // Pass a pointer to the value of interest.
// In this case using &.
// T3 -- B() has used its pointer to change the value of interest
}
I guess that covers the Vector class.
If you need to know more about it, you're welcome to read the API specs for it. I also
greatly encourage you to look at java.util.Vector source, and see for
yourself what's going on inside that incredibly simple structure.
Back to Table of Contents
A D V E R T I S E M E N T
|
Subscribe to SourceCodesWorld - Techies Talk |
|